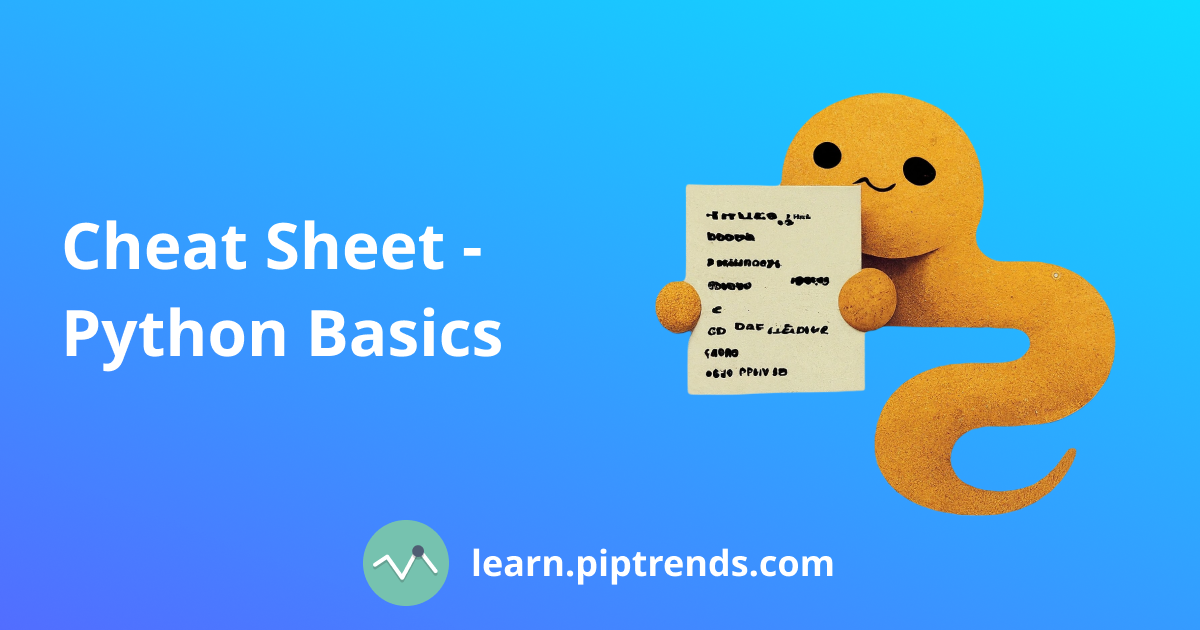
Python Basics
A Python cheatsheet covering variables, data types, control structures, functions, file operations, classes, exceptions handling, modules, and virtual environments, with concise explanations and examples for all skill levels.
Author: Ayush Jain | Date: 2024-04-01
Variables and Data Types
Lists
my_list = [1, 2, 3, 4, 5]
# Accessing elements
print(my_list[0]) # Output: 1
# Slicing
print(my_list[1:3]) # Output: [2, 3]
# List methods
my_list.append(6)
my_list.pop()
Control Structures
If-Else
Loops
For Loop
While Loop
Functions
File Operations
# Writing to a file
with open("example.txt", "w") as f:
f.write("Hello, World!")
# Reading from a file
with open("example.txt", "r") as f:
content = f.read()
print(content)
Classes
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def display_info(self):
print(f"{self.make} {self.model}")